These questions and answers have been reviewed and verified by Dmytro Herasymuk, Lead Software Engineer and Certified Technical Interviewer at EPAM Anywhere. Thanks a lot, Dmytro!
Preparing for a job interview can be a tiresome task, especially when it comes to technical roles like a Ruby on Rails developer. Whether you're interviewing for on-site or remote Ruby on Rails developer jobs, you'll likely face a series of challenging questions designed to assess your understanding and proficiency in Ruby on Rails. This guide presents a comprehensive list of Ruby on Rails developer interview questions, providing you with the insights needed to showcase your skills and land your dream job. Let's dive in and start preparing for your upcoming interview.
tired of job hunting?
Scroll no more. Send us your CV and we'll match it with our best Ruby on Rails developer jobs for you.
1. What is Ruby on Rails?
Ruby on Rails is a server-side web applications framework written in Ruby. It adheres to the Model-View-Controller (MVC) architectural pattern and has default structures for web pages, web services, and a database. It encourages and simplifies web standards such as JSON or XML for data transfer along with HTML, CSS, and JavaScript for user interface. Additionally, Rails highlights the importance of other well-known engineering patterns and paradigms like convention over configuration (CoC), don't repeat yourself (DRY), and the active record pattern.
2. Can you explain the MVC architecture of Rails?
MVC stands for Model-View-Controller. A software design pattern separates the application into three interconnected components. This separation results in user requests being processed more efficiently.
- Model: The Model corresponds to all the data-related logic. It interacts with the database and defines the schema and relationships between the tables. It represents the data and the rules to manipulate that data.
- View: The View is used for the UI logic of the application. It represents the application's presentation layer. It's responsible for rendering data in a human-readable format.
- Controller: The Controller is an interface found between the Model and the View. It processes all the incoming requests, interacts with the model to fetch data, and passes the data to the view for rendering.
In Rails, when a user sends a request, it first reaches the Controller, which interacts with the Model to fetch data. The Controller then passes this data to the View. The View renders the data in a human-readable format and sends it back to the user.
3. What is the role of the 'yield' keyword in Ruby?
In Ruby, the `yield` keyword is used within a method to pause its execution and pass control to a block provided when the method is called. The method can then resume execution once the block has completed. This allows for greater flexibility and code reuse, as the same method can perform different actions depending on the block passed to it.
`Yield` can also pass parameters to the block, allowing for dynamic interaction between the method and the block's logic. It's a fundamental part of Ruby's approach to blocks, enabling the creation of customizable and maintainable code structures. The presence of a block can be checked with `block_given?`, allowing methods to yield to a block if one is provided optionally. This underpins the implementation of iterators, callbacks, and other block-based patterns in Ruby, making `yield` a key aspect of Ruby's expressive and flexible programming style.
4. What is Active Record in Rails?
Active record is the M in MVC (the model) which is the system layer responsible for representing business data and logic. It's an implementation of the Active Record design pattern, which describes an Object-Relational Mapping (ORM) system.
Active Record in Rails provides an abstraction over the SQL database and allows you to interact with your database, like retrieving, inserting, and manipulating data, in a very intuitive and Ruby-like manner. It translates your object-oriented models into table-oriented relational algebra without you having to write SQL queries.
Active Record also offers a series of advanced features like data validation, associations between models (like one-to-many or many-to-many), and advanced query capabilities. It's a fundamental component of Rails that significantly simplifies the code needed for database communication.
5. What default Rake tasks do you use in Rails?
Rake is a task management utility in Ruby. It allows you to define tasks and describe dependencies and group tasks in a namespace.
In Rails, Rake is used to automate a number of developer tasks. Rails provides several Rake tasks out of the box, including:
- Database tasks: These tasks allow you to create, drop, migrate, and seed your database. For example, `rake db:migrate` is used to run database migrations.
- Test tasks: Rails provides tasks for running tests, like `rake test` to run all tests, or `rake test:models` to run just model tests.
- Asset tasks: These tasks help manage assets, like `rake assets:precompile` to precompile assets for deployment to production.
- Log tasks: For example, `rake log:clear` can be used to clear all log files.
You can also define your own custom Rake tasks for any scripts or procedures you need to automate in your development process. Rake tasks can be defined in any file under the `lib/tasks` directory with a `.rake` extension.
6. What is a Gem in Ruby?
A Gem is a module or a package in Ruby. It provides a specific functionality which can be used in other programs. Rails itself is a gem. Other examples of gems include Devise for authentication, Paperclip for file attachment, etc.
7. What is REST and how does Rails follow it?
REST (Representational State Transfer) is a software architectural style defining constraints for creating web services. In Rails, REST is a standard convention for building web applications.
A RESTful Rails application is built around the concept of resources, which are the objects your application provides to users. Each resource corresponds to a specific URL, and can be manipulated using standard HTTP verbs (GET, POST, PUT, DELETE, etc.).
For example, if you have a resource called `articles`, you might have the following routes in a RESTful Rails application:
- `GET /articles` to list all articles
- `POST /articles` to create a new article
- `GET /articles/:id` to show a specific article
- `PUT /articles/:id` to update a specific article
- `DELETE /articles/:id` to delete a specific article
Rails provides built-in support for creating RESTful applications, including routing, controllers, and views.
8. What is CSRF? How does Rails protect against it?
CSRF stands for Cross-Site Request Forgery. It's an attack that tricks the victim into submitting a malicious request. Rails includes a built-in security feature to protect from CSRF attacks by including a security token in all forms and Ajax requests generated by Rails. The session will be reset if the security token doesn't match what was expected.
9. Explain the difference between a String and a Symbol in Ruby
In Ruby, both Strings and Symbols can represent text, but they serve distinct purposes and have different behaviors under the hood.
String: A String in Ruby is mutable, meaning it can be altered after it has been created. Strings are typically used to manipulate text data, such as names, sentences, or any sequence of characters that need to be changed or processed. Since Strings are mutable, Ruby allocates a new object in memory each time you create a string, even if the string value is identical to an existing one. This behavior can increase memory usage if many duplicate Strings are created.
```ruby string1 = "example" string2 = "example" string1.object_id != string2.object_id # Evaluates to true ``` |
Symbol: A Symbol, denoted by a colon (`:`) followed by a name, is immutable and unique. Once a Symbol is created, it is not duplicated. Ruby reuses the object for that Symbol, meaning if you reference the same Symbol multiple times throughout your program, all of those references point to the same object in memory. Due to their immutability and uniqueness, Symbols are more memory-efficient than Strings. They are commonly used as identifiers, keys in hashes, or method names.
symbol1 = :example symbol2 = :example symbol1.object_id == symbol2.object_id # Evaluates to true |
10. What is the difference between 'render' and 'redirect_to' in Rails?
'render' and 'redirect_to' serve two different purposes. 'render' is used when you want to show the user a new page without moving to a new URL. 'redirect_to' is used when you want to send the user to a new URL.The 'redirect_to' responds with 301 and trigger one more browser request to request the new URL.
11. What is a migration in Rails?
A migration in Rails is a tool for changing the database schema over time. Instead of writing SQL directly, you define a set of changes in a Ruby DSL (domain-specific language). This makes migrations database-agnostic and version-controlled.
Migrations allow you to alter your database structure in a structured and organized manner, effectively implementing version control at the database level. You can use migrations to create or drop tables, change the data type of columns, rename columns, add or remove indexes, and much more.
Each migration is a new 'version' of the database. Rails keeps track of the run migrations, so it can roll back changes and apply new ones as needed. This makes updating your database schema a repeatable process and helps keep your database schema in sync with your application code.
12. What is the asset pipeline in Rails?
The asset pipeline in Rails is a framework that provides features to compress and concatenate JavaScript, CSS, and image assets. It allows you to write these assets in other languages such as CoffeeScript, Sass, and ERB.
The asset pipeline makes your application's assets faster to download and easier to manage by enabling features such as:
- Asset compression: It reduces the size of CSS and JavaScript files, which makes your application faster to download and load.
- Asset concatenation: It combines all your CSS files into one stylesheet and all your JavaScript files into one script, reducing the number of requests a client's browser needs to make.
- Preprocessing: It allows coding assets via higher-level languages like CoffeeScript, Sass, and ERB.
- Fingerprinting: It appends a hash to filenames, which aids in cache busting when a file is updated.
The asset pipeline is enabled by default starting from Rails 3.1. Rails uses the `sprockets-rails` gem, a wrapper around the `sprockets` gem for managing assets.
13. What is the difference between 'has_many' and 'belongs_to' associations in Rails?
In Rails, `has_many` and `belongs_to` associations define different sides of database relationships between models.
- `belongs_to` is used in a model to define a one-to-one relationship towards another model, indicating that this model is the "child". It implies that the model where `belongs_to` is declared contains the foreign key. For example, in a `Comment` model that `belongs_to :post`, the comments table has a `post_id` column linking it to its parent post.
- `has_many` declares a one-to-many relationship from one model to another, stating that a single instance of this model can be associated with many instances of another model. The model declaring `has_many` holds no foreign key. Instead, the associated model contains the foreign key pointing back. For instance, a `Post` model having `has_many :comments` indicates that each post can have multiple comments.
The key difference lies in the direction and type of relationship: `belongs_to` sets up a child's link to its parent (requires foreign key in the child), while `has_many` sets up a parent's link to its children (foreign key in the children).
14. What is the purpose of modules in Rails?
Modules in Rails serve several purposes. They are used to group related methods, constants, and classes together and provide two major benefits:
- Namespacing: Modules create a namespace for methods, classes, and constants to avoid naming collisions. For example, Rails provides several modules such as `ActiveRecord` and `ActionPack`, each encapsulating a related set of functionality.
- Mixins: Modules allow for the creation of mixins. You can define a set of methods in a module and then include that module in a class using the `include` method. The class will then have access to the methods, essentially using the module as a mixin.
In addition, modules can also be used to create custom exceptions and extend other modules or classes, adding new methods to them. They are a fundamental part of structuring code in Ruby and Rails applications.
15. What is the difference between a class and a module in Ruby?
The main difference between a class and a module in Ruby lies in their usage and functionality:
- Class: A class is a blueprint from which individual objects are created. It defines attributes and methods that each object of the class will have. A class can be instantiated, allowing for inheritance, meaning you can create a subclass that inherits all the characteristics of its superclass.
- Module: A module in Ruby is a collection of methods, constants, and class variables. Modules are defined much like classes, but they can't be instantiated or subclassed or have instances. Instead, you can mix a module into a class or another module using the `include` method, essentially using it as a mixin to add additional behavior to the class.
In summary, you would use a class to create objects with similar behavior (instances of the same class), and use a module when you want to group functionalities and include them into other classes as needed.
16. What is the purpose of 'self' in Ruby?
In Ruby, `self` refers to the current object within a code block. Its specific meaning varies depending on the context in which it's used:
1. Within instance methods: `self` points to the instance of the class that is executing the current method. This allows you to call other instance methods, access instance variables, or set properties on the instance.
class Example attr_accessor :name def initialize(name) @name = name end def update_name(new_name) self.name = new_name # self refers to the instance end |
2. Within class methods: When used inside class methods, `self` refers to the class itself, allowing you to define or call other class methods or access class variables from within.
class Example @class_variable = 10 def self.class_method @class_variable # self refers to the class end |
3. Within class or module definitions, but outside any method: Here, `self` refers to the class or module being defined. This is often used when declaring class methods or class/module variables.
class Example def self.class_method # Method definition where self refers to the class end |
The purpose of `self` is to provide a clear and explicit way to reference the current object or class, enhancing code readability and maintainability by making it clear whether you're dealing with instance-level or class-level data and behavior.
17. What is the difference between 'nil?', 'empty?', and 'blank?' in Ruby on Rails?
In Ruby on Rails, 'nil?', 'empty?', and 'blank?' are methods that can be used to check if an object has a value or not, but they work in slightly different ways:
- 'nil?': This is a standard Ruby method that can be called on all objects. It returns true if the object is `nil`, and false otherwise.
- 'empty?': This method is mainly used on arrays, hashes, and strings. It returns true if these objects contain no elements or if a string length is zero. It will raise an error if used on `nil`.
- 'blank?': This is a Rails method that can be used on any object. It returns true if the object is `nil`, empty, or a whitespace string. For example, it will return true for `nil`, `[]`, `{}`, `""`, `" "`, etc.
In summary, 'nil?' is used to check if an object is `nil`, 'empty?' is used to check if an object has no elements or characters, and 'blank?' is a more general check for whether an object is `nil`, empty, or a whitespace string.
18. What is the use of 'respond_to' in Rails controllers?
'respond_to' is used in Rails controllers to specify what kind of content the method will respond with, based on the HTTP headers in the request. It allows your application to serve different types of content (like HTML, XML, JSON, etc.) from the same controller action, depending on the client's preferred format.
Here's an example of how 'respond_to' might be used in a controller action:
def show @user = User.find(params[:id]) respond_to do |format| format.html # show.html.erb format.json { render json: @user } end end |
In this example, if the client requests HTML (or doesn't specify a format), Rails will render the `show.html.erb` view. If the client requests JSON, Rails will render the user data as JSON.
'respond_to' is a key part of building RESTful applications with Rails, allowing the same resources to be accessed in different formats for different purposes.
19. What is the difference between 'PUT' and 'PATCH' in Rails?
'PUT' and 'PATCH' are HTTP verbs used to update resources in a RESTful system, and Rails routes can be configured to respond to both. However, there's a key difference in how they are intended to be used:
- 'PUT' is idempotent, meaning it should always result in the same resource state. It's used when you want to update the entire resource with a completely new set of data. If you send the same 'PUT' request multiple times, the result should always be the same final resource state.
- 'PATCH', on the other hand, is used when you want to make a partial update to the resource, like changing just one field. It's not necessarily idempotent, as multiple identical 'PATCH' requests may have different results if applied more than once.
In Rails, both 'PUT' and 'PATCH' map to the `update` action in the controller, but the way you handle the incoming parameters in the `update` action may differ based on whether you're expecting a full or partial update.
20. What is the purpose of 'before_action' in Rails?
'before_action' is a filter provided by Rails. It's a method that gets called before any controller action is performed. It's useful for a variety of purposes, such as:
- Authentication: You can use a 'before_action' to check if a user is logged in before letting them access some actions.
- Loading a resource: If several actions need to work with the same database resource, you can use a 'before_action' to load the resource and make it available to all the actions.
- Redirecting: If you need to redirect the request under certain conditions, you can use a 'before_action' to do it before the controller action is run.
You can specify which actions the 'before_action' applies to with the `:only` or `:except` options. For example, `before_action:authenticate_user!, only: [: edit, : update]` will run the `authenticate_user!` method before the `edit` and `update` actions.
Ruby on Rails senior developer interview questions
21. How does Rails implement AJAX?
Rails uses the `remote: true` option to enable AJAX. This option can be used with form helpers and `link_to`. When this option is set, Rails will use unobtrusive JavaScript to send an AJAX request instead of a regular regular HTTP request.
22. What is the role of Rack in Rails?
Rack provides a minimal, modular, and adaptable interface for developing web applications in Ruby. It abstracts the common API of HTTP servers, allowing Rails to interface with many different web servers easily.
23. How does Rails handle database transactions?
Rails provides a simple API for handling transactions. You can start a transaction with `ActiveRecord::Base.transaction` and perform database operations inside a block. Rails will roll back the transaction if any exception is raised within the block.
24. What is the purpose of the `responders` gem in Rails?
The ‘responders’ gem in Rails is a collection of Rails responders, objects that handle the behavior of controller actions. It aims to simplify the creation of custom responders by providing reusable behavior.
This gem provides a set of ‘responders’ modules to dry up your Rails 4.2+ app. It introduces a responder for flash messages, HTTP cache and others. It also provides a generator for you to create your custom responders.
For example, the FlashResponder sets the flash message based on the controller action and the resource status. The HttpCacheResponder handles HTTP cache, returning 304 status when the resource is not modified.
By using the responder's gem, you can make your controllers DRYer and more consistent.
25. How does Rails handle internationalization (i18n)?
Rails provides a simple and flexible set of APIs for handling internationalization. The process involves abstracting all the strings in your application into a separate file, which you can then translate into different languages.
Here's how it works:
- You store translations in YAML files, one for each language, under the `config/locales` directory. The translations are stored as key-value pairs, where the key is a symbol you'll use to reference the translation, and the value is the translated string.
- You use the `I18n.t` method (or its shorthand version, `t`) in your views, controllers, and models to replace hard-coded strings with references to your translations.
- You set the current locale with `I18n.locale = :es` (for Spanish, for example), and Rails will use that locale for all translations until you change it.
Rails' internationalization system also supports pluralization, interpolation, and localization of dates, times, and numbers. It's a powerful tool for making your application accessible to users worldwide.
26. What is the role of Active Job in Rails?
Active Job is a framework for declaring jobs that various queuing backends can run. These jobs are units of work that can be performed outside of the usual request-response cycle, allowing long-running tasks to be performed without tying up the web server.
Active Job provides a common interface for creating background jobs, allowing you to switch queuing backends easily. It has built-in queuing adapters for multiple queuing systems, including Sidekiq, Resque, Delayed Job.
With Active Job, you can schedule tasks to run asynchronously, at a set time in the future, or even on a recurring schedule. It also provides hooks for callbacks when a job is enqueued, performed, or encounters an error.
27. How does Rails handle caching?
Caching is a technique Rails uses to speed up content delivery by storing the results of expensive or time-consuming operations and reusing them in subsequent requests. Rails provides several mechanisms for caching:
- Page caching: This is a Rails mechanism that allows the request for a generated page to be fulfilled by the webserver (i.e., Apache or NGINX), without ever having to go through the Rails stack. It's super-fast but has been removed from Rails 4 due to its complexity.
- Action caching: This works like Page Caching, except the incoming web request hits the Rails stack so that filters can be run on it before the cache is served. This allows authentication and other restrictions to be run before serving the cache.
- Fragment caching stores a block or fragment of the view to prevent costly database queries and render calls. It can be used to cache various parts of your application's views.
- SQL caching: This caches the result set returned by each query so that it hits the cache instead of the database if the same query is returned in the same request.
- Low-level caching: Rails also supports low-level caching in your application, allowing great flexibility in caching.
Rails use the `ActiveSupport::Cache::Store` module for interacting with the caching system, allowing different cache stores to be used interchangeably.
28. What is the purpose of the `turbolinks` gem in Rails?
The `turbolinks’ gem is designed to make navigating your web application faster. It improves the perceived performance of your application by speeding up page loads.
Here's how it works: instead of letting the browser recompile the JavaScript and CSS between each page change, it keeps the current page instance alive and replaces only the body and the title in the head. This gives you the speed of a single-page web application without having to write your application as a single-page web application.
When you click on a link, turbolinks automatically fetches the page, swaps in its `<body>`, and merges its `<head>`, all without incurring the cost of a full page load.
It's important to note that turbolinks can lead to some complexities, especially when dealing with JavaScript that's designed to run on a full page load. However, it can significantly improve performance when used correctly.
29. How does Rails handle file uploads?
Rails can handle file uploads using the Active Storage framework. Active Storage assists in uploading files to a cloud storage service like Amazon S3, Google Cloud Storage, or Microsoft Azure Storage, and attaching those files to Active Record objects.
Here's a basic example of how it works:
1. In your form, you would include a file field:
<%= form_with model: @user do |form| %> <%= form.file_field :avatar %> <% end %> |
2. In your model, you would declare that the model has an attached file:
class User < ApplicationRecord has_one_attached :avatar end |
3. In your controller, you would permit the file parameter:
params.require(:user).permit(:username, :avatar) |
4. You can then display the uploaded file in your view:
<%= image_tag @user.avatar %> |
Active Storage provides a lot of flexibility for handling file uploads, including support for direct uploads to the cloud, generating image variants, and creating previews of non-image files.
30. What is the role of Webpacker in Rails?
Webpacker is a wrapper for the Webpack module bundler. It's a tool that helps you manage JavaScript, CSS, and other assets in your Rails application.
Webpacker makes it easy to use Webpack in your Rails application to bundle and manage assets. It allows you to use the latest JavaScript features, including modules, and supports other assets like CSS, images, and fonts.
Here are some of the things you can do with Webpacker:
- Organize your JavaScript code into modules, which can import and export functions and objects from and to other modules.
- Use modern JavaScript features by leveraging Babel, a JavaScript compiler.
- Easily integrate with npm to manage JavaScript libraries and dependencies.
- Use loaders to process other types of files, like CSS, SCSS, images, and fonts.
- Optimize your assets for production with minification, tree shaking, and other techniques.
Starting from Rails 6, Webpacker is the default JavaScript compiler for Rails, replacing the asset pipeline for JavaScript management, although the asset pipeline is still used for other types of assets.
31. How does Rails handle real-time features?
Rails can handle real-time features with Action Cable, a framework for handling WebSockets. WebSockets provide a persistent connection between the client and the server, enabling real-time features like chat, notifications, live updates, and more.
Action Cable integrates WebSockets with the rest of your Rails application. It allows you to write real-time features in the same style and form as the rest of your application. Here's a basic example of how it works:
1. You define a channel, which is a logical unit of work similar to a controller in a regular MVC setup.
class ChatChannel < ApplicationCable::Channel def subscribed stream_from "chat_#{params[:room]}" end def receive(data) ActionCable.server.broadcast("chat_#{params[:room]}", data) end end ``` |
2. On the client side, you subscribe to the channel and define how to handle incoming data:
App.chat = App.cable.subscriptions.create({ channel: "ChatChannel", room: "room1" }, { received: function(data) { // Handle incoming data } }); |
3. You can then broadcast data to the channel from anywhere in your Rails application:
```ruby ActionCable.server.broadcast("chat_room1", message: "Hello, world!") |
This is a simple example, but Action Cable is capable of much more, including broadcasting updates to multiple users, tracking user presence, and more. It's a powerful tool for adding real-time features to your Rails application.
32. What is the purpose of the `spring` gem in Rails?
The `spring` gem is a Rails application preloader. It is designed to speed up development by running your application in the background, so that there is no need to boot it when you run a test, rake task or migration.
Here's how it works: when you run a command that has been springified (like `rails console` or `rspec`), Spring loads a copy of your application into memory and keeps it running in the background. Then, when you run the command, it uses the already-loaded application, so it doesn't have to boot the application again.
This can significantly speed up common development tasks. For example, running a single test or a rake task can be much faster with Spring, because you don't have to wait for the entire Rails environment to load.
Spring is included by default in new Rails applications (from Rails 4.1 onwards). It's designed to be unobtrusive and to stay out of your way unless you explicitly use it.
33. How does Rails handle API versioning?
Rails doesn't provide a built-in way to handle API versioning, but it can be easily implemented using routing constraints or request headers.
34. What is the role of the `puma` gem in Rails?
The `puma` gem is a Ruby web server built for speed and parallelism. It's designed to handle concurrent connections in a multi-threaded environment, making it a good choice for any Rails application that expects to handle a large number of incoming requests at the same time.
Puma works by creating a new thread for each incoming request, and can handle many requests concurrently. This makes it more efficient than single-threaded servers, especially for applications that make heavy use of I/O operations or external APIs.
Puma also supports "clustered mode" for multi-process environments, and "hybrid mode" which combines both multi-thread and multi-process.
Starting from Rails 5, Puma is the default web server for Rails in development and production. It's a robust, fast, and concurrent server, making it a great choice for running Rails applications.
35. How does Rails handle security?
Rails has a number of built-in features to handle security and help protect your application against common web attacks:
- Cross-site scripting (XSS) protection: Rails automatically escapes any data that is output in view templates, protecting against XSS attacks.
- Cross-site request forgery (CSRF) protection: Rails includes a CSRF protection mechanism which generates and checks a unique token for each form that is submitted.
- SQL injection protection: Active Record uses parameterized queries to ensure that all data sent to the database is properly escaped, protecting against SQL injection attacks.
- Secure cookies: Rails can store session data in cookies which are signed and/or encrypted to prevent tampering.
- HTTP strict transport security (HSTS): Rails can be configured to use HSTS, which tells the browser to only connect to your application over a secure connection.
- Content security policy (CSP): Rails 5.2 introduced support for creating and managing a content security policy for your application, helping to mitigate cross-site scripting and other content injection attacks.
- Strong parameters: This feature ensures that only the necessary attributes can be updated in the database, protecting against mass assignment vulnerabilities.
In addition to these features, Rails also provides a secure password hashing system via Active Model Secure Password, and has a number of guides and resources available to help developers understand and mitigate common web security threats.
36. What is the purpose of the `byebug` gem in Rails?
The `byebug` gem is a simple-to-use, feature-rich debugger for Ruby. It uses the TracePoint API for execution control and the Debug Inspector API for call stack navigation, so it doesn't depend on internal core sources.
37. How does Rails handle email sending?
Rails handles email sending with the Action Mailer framework. Action Mailer allows you to send emails from your application using mailer classes and views.
Here's a basic example of how it works:
1. You create a mailer class, which is similar to a controller:
class UserMailer < ApplicationMailer def welcome_email(user) @user = user mail(to: @user.email, subject: 'Welcome to My Awesome Site') end end |
2. You create a view for the email, just like you would for a regular controller action:
<!-- app/views/user_mailer/welcome_email.html.erb --> Welcome, <%= @user.name %>! |
3. You can then send the email from anywhere in your application:
UserMailer.welcome_email(@user).deliver_now |
Action Mailer integrates with a number of delivery methods, including SMTP, Sendmail, and others. It also supports advanced features like attachments, multipart messages, and custom headers.
38. What is the role of the `sprockets` gem in Rails?
The `sprockets` gem is a Rack-based asset packaging system that concatenates and serves JavaScript, CoffeeScript, CSS, LESS, Sass, and SCSS.
39. How does Rails handle background jobs?
Rails can handle background jobs with the `ActiveJob` framework. It provides a way to run jobs asynchronously, outside of the request-response cycle.
40. What is the purpose of the `brakeman` gem in Rails?
The `brakeman` gem is a static analysis security vulnerability scanner for Ruby on Rails applications. It scans Rails applications and produces a report of all security issues it has found.
Conclusion
In conclusion, preparing for Ruby on Rails developer interview questions is a crucial step towards landing your dream job. Whether you're an experienced engineer or just starting out, these questions will equip you with the knowledge needed to impress potential employers. If you're seeking remote opportunities, consider applying for a Ruby on Rails developer position at EPAM Anywhere. By understanding these interview questions, you'll be one step closer to securing a remote position as a Ruby on Rails developer with us. Best of luck with your interview!
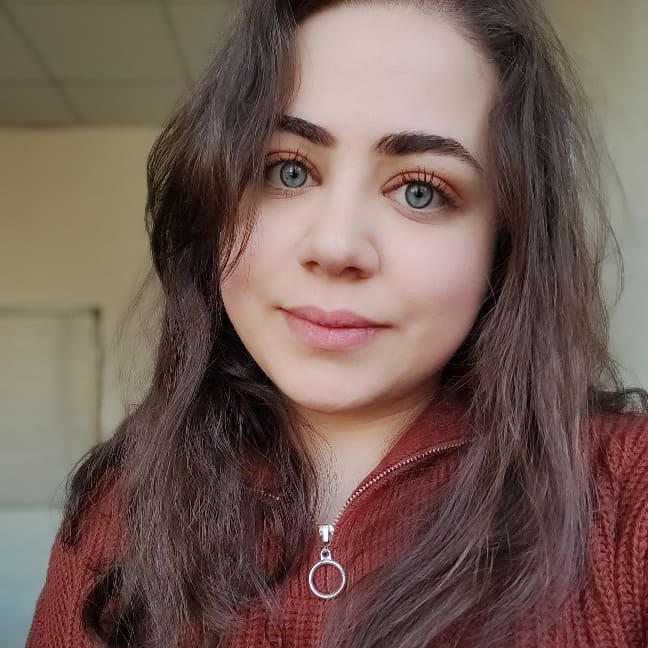
With a focus on remote lifestyle and career development, Gayane shares practical insight and career advice that informs and empowers tech talent to thrive in the world of remote work.
With a focus on remote lifestyle and career development, Gayane shares practical insight and career advice that informs and empowers tech talent to thrive in the world of remote work.
Explore our Editorial Policy to learn more about our standards for content creation.
read more